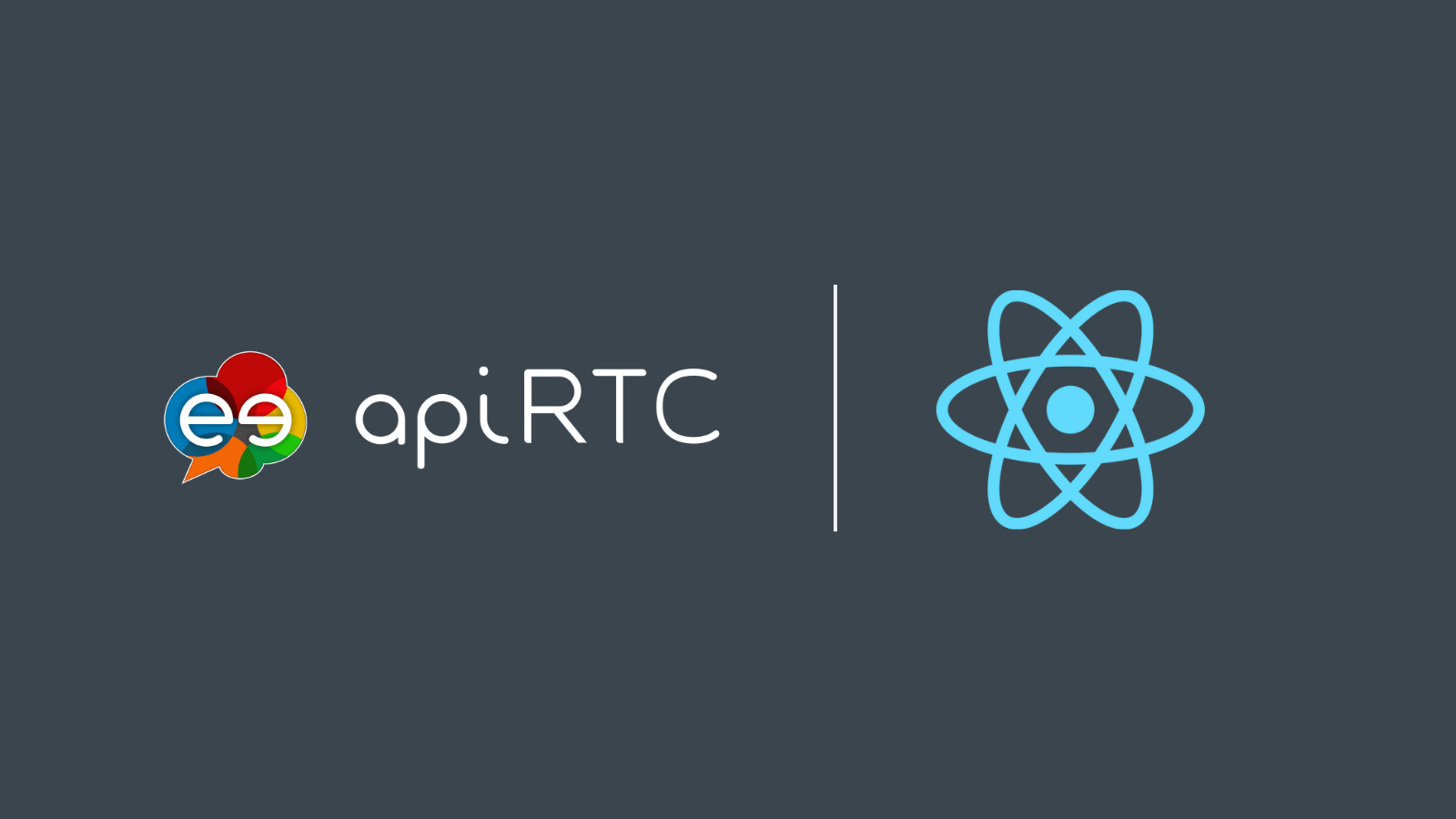
2-step tutorial to build a video chat in ReactJS with the ApiRTC ReactJS Hooks library
Last Updated on January 30, 2023
You were thinking ApiRTC.js was an easy thing to play with? Gain 10x development time by using the ApiRTC React Hooks Library, and integrate video communication in your app that reach enterprise-grade needs: stability, quality of service and integration into an existing ecosystem.
Table of Contents
What is a ‘hook’ in ReactJS?
A hook is basically a module that makes state management of an object easier and well integrated within the ReactJS rendering lifecycle. Although High-Order Components and component.props can implement the same logic, it needs a lot more components and props to be managed.
Hooks simplifies the use of functional logic into your app.
You may know the useState()
standard hook that enables to make a variable stateful and integrated into the ReactJS lifecycle.
ApiRTC implements the video communication lifecycle into Hooks modules, gathered into one library available as the @apirtc/react-lib
package on NPM.
What can I do with the ApiRTC ReactJS hooks?
ApiRTC ReactJS Hooks enables any developer to:
- Create or join a Conversation
- Grab the local devices streams, display the local video and publish them into a Conversation
- Listen to the remote streams and display them onto a web page
- Apply noise reduction on audio and blur or image in the background of the video stream
- Manage groups of connected participants (presence management)
- Apply moderation functions in the Conversation
- Send & Receive chat messages
See the ApiRTC React library’s README file for detailed information on available hooks and components.
Setting up your development environment
Prerequisite
You need a Linux environment with the following installed:
- NodeJS in its latest version
- NPM in its latest version
The best way to get you machine set up is to use the nvm installer for both nodeJS and NPM:
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.39.3/install.sh | bash nvm install node
Create a ReactJS app boilerplate
Create a new ReactJS-enabled web app by using create-react-app
command:
npx create-react-app myApiRTCReactApp cd myApiRTCReactApp
Install ApiRTC libs
Use NPM to install ApiRTC needed library:
npm install @apirtc/apirtc @apirtc/react-lib
You are all set for building your first ReactJS-based video communication app, well done!
Add the video communication feature to the ReactJS app
This app will simply connect to a Conversation named conversationName
and will share the video streams across the participants thanks to 4 hooks:
useSession
: connect to the ApiRTC serviceuseCameraStream
: grab the local video stream coming from the camerauseConversation
: join a Conversation and get the always-updated state of ituseConversationStreams
: get an updated list of Streams flowing through the Conversation
Additionnaly, we will use the VideoStream
component that makes video display easier.
Replace the /src/App.js file content by the following:
import { useSession, useConversation, useCameraStream, useConversationStreams, VideoStream } from '@apirtc/react-lib' import './App.css'; function App() { //Get a connection session to ApiRTC Video platform const { session } = useSession({ apiKey: 'INSERT_YOUR_API_KEY_HERE' }); // Get an account on cloud.apirtc.com and retrieve your Api Key. //Grab the local camera video and audio stream const { stream: localStream } = useCameraStream(session); // Get the conversation stateful object const { conversation } = useConversation(session, 'conversationName', undefined, true); // Get the list of streams exchanged in the conversation const { publishedStreams, subscribedStreams } = useConversationStreams(conversation, localStream ? [{ stream: localStream }] : []); return ( <div className="App"> <header className="App-header"> <div id="mememe-container"> <h2>Local Streams</h2> { localStream && <VideoStream stream={localStream} muted={true}></VideoStream> } </div> <div id='remote-streams-container'> <h2>Remote Streams</h2> { subscribedStreams.map( (stream, index) =>{ return (<VideoStream stream={stream} muted={false} key={index}></VideoStream>) }) } </div> </header> </div> ); } export default App;
Replace INSERT_YOUR_API_KEY_HERE
by your actual API Key. You can have one (free) on https://cloud.apirtc.com.
Source Code
The source code of this getting started article is available on the dedicated GitHub repository, in the /react-hooks-basic
folder.
Read more
Continue to explore ApiRTC:
- Get to know the video communication lifecycle
- Understand the apirtc-architecture.md
- Read the README file of the ApiRTC React Lib to get more details on what is possible